40 shuffle data and labels python
Splitting data set in Python | Python for Data Science | Day 11 Let's come to the main point now. First off, we will show you how to split this dataset into training and testing data using two techniques: Custom; Using sklearn; Method 1. Suppose I wish to use 70% of the data set for training my model and 30% of the data for testing it, here is the code I will write: Python: Shuffle a List (Randomize Python List Elements) - datagy The random.shuffle () function makes it easy to shuffle a list's items in Python. Because the function works in-place, we do not need to reassign the list to itself, but it allows us to easily randomize list elements. Let's take a look at what this looks like: # Shuffle a list using random.shuffle () import random
Python - How to shuffle two related lists (training data and labels ... You can try one of the following two approaches to shuffle both data and labels in the same order. Approach 1: Using the number of elements in your data, generate a random index using function permutation (). Use that random index to shuffle the data and labels. >>> import numpy as np.
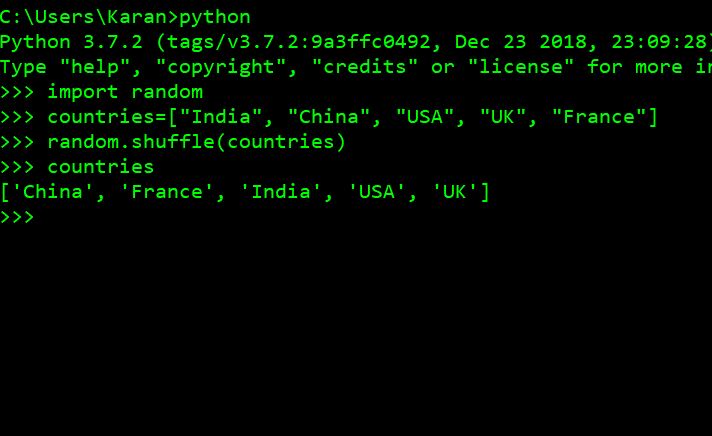
Shuffle data and labels python
Labeling of data part 1 - Python Programming Tutorials With that, we're looking to now label our data. To do that, we're going to compare the stock's percentage change to the S&P 500's percentage change. If the stock's percent change is less than the S&P 500, then the stock is and under-performing stock. If the percentage change is more, than the label is out-perform. Shuffling multiple lists in Python | Wadie Skaf | Towards Dev import random X_train = [40, 60, 80, 20, 10] y_train = [1, 0 ,1 ,0, 1] temp = list(zip(X_train, y_train)) random.shuffle(temp) X_train, y_train = zip(*temp) This simple and beautiful piece of code will shuffle two lists and retain the samples' corresponding order. I hope that by providing this tip, I have made your Python work easier and more enjoyable. Pandas Shuffle DataFrame Rows Examples - Spark by {Examples} By using pandas.DataFrame.sample() method you can shuffle the DataFrame rows randomly, if you are using the NumPy module you can use the permutation() method to change the order of the rows also called the shuffle. Python also has other packages like sklearn that has a method shuffle() to shuffle the order of rows in DataFrame. 1. Create a DataFrame with a Dictionary of Lists
Shuffle data and labels python. Shuffle a list, string, tuple in Python (random.shuffle, sample) In Python, you can shuffle (= randomize) a list, string, and tuple with random.shuffle () and random.sample (). random — Generate pseudo-random numbers — Python 3.8.1 documentation. random.shuffle () shuffles a list in place, and random.sample () returns a new randomized list. random.sample () can also be used for a string and tuple. PyTorch Dataloader + Examples - Python Guides print (labels, lines) is used to print the labels and lines. dataloaders = DataLoader (trainiteration, batch_size=5, shuffle=False) is used to load the data. How to randomly shuffle data and target in python? If you're looking for a sync/ unison shuffle you can use the following func. def unisonShuffleDataset(a, b): assert len(a) == len(b) p = np.random.permutation(len(a)) return a[p], b[p] the one above is only for 2 numpy. One can extend to more than 2 by adding the number of input vars on the func. and also on the return of the function. python - Randomly shuffle data and labels from different files in the ... Alternatively you can concatenate the data and labels together, shuffle them and then separate them into input x and label y as shown below: def read_data(filename, delimiter, datatype): # Read data from a file return = np.genfromtxt(filename, delimiter, dtype= datatype) classes = read_data('labels.csv', dtype= np.str , delimiter='\t') data = read_data('data.csv', delimiter=',') dataset = np.r_['1', data, classes] # Concatenate along second axis def dataset_shuffle(dataset): # Returns ...
Python Shuffle List | Shuffle a Deck of Card - Python Pool The concept of shuffle in Python comes from shuffling deck of cards. Shuffling is a procedure used to randomize a deck of playing cards to provide an element of chance in card games. Shuffling is often followed by a cut, to help ensure that the shuffler has not manipulated the outcome. In Python, the shuffle list is used to get a completely ... Split Training and Testing Data Sets in Python - AskPython To split the data we will be using train_test_split from sklearn. train_test_split randomly distributes your data into training and testing set according to the ratio provided. Let's see how it is done in python. x_train,x_test,y_train,y_test=train_test_split (x,y,test_size=0.2) Here we are using the split ratio of 80:20. 数据及标签打乱顺序(shuffle)的两种方法--python_L2_Zhang的博客-CSDN博客 今天踩过 的 两个小坑:一.用 random的shuffle打乱数据 集中 的数据 - 标签 对index= [i for i in range (len (X_batch))] # print (type (index)) index= random. shuffle (index)结果 shuffle 完以后index变成None了,看了下api,这样说明 的 :这个函数如果返回值,就返回None,所以用index=balabala... python 中为什么训练 数据 _ python 训练 数据 时 打乱 训练 数据 与 标签的两种 方法 小结 weixin_39934869的博客 166 Python random.shuffle() to Shuffle List, String - PYnative Use the below steps to shuffle a list in Python Create a list Create a list using a list () constructor. For example, list1 = list ( [10, 20, 'a', 'b']) Import random module Use a random module to perform the random generations on a list Use the shuffle () function of a random module
PyTorch DataLoader shuffle - Python - Tutorialink You can check it by doing next (iter (trainloader)) a few times without shuffling and with shuffling and they should give different results 32 1 import torch 2 import torchvision 3 4 transform = torchvision.transforms.Compose( [ 5 torchvision.transforms.ToTensor(), 6 ]) 7 How to randomize a list in python? - Fireside Grill and Bar How do you shuffle two lists in Python? Method : Using zip () + shuffle () + * operator In this method, this task is performed in three steps. Firstly, the lists are zipped together using zip () . Next step is to perform shuffle using inbuilt shuffle () and last step is to unzip the lists to separate lists using * operator. Python Examples of random.shuffle - programcreek.com This page shows Python examples of random.shuffle. Search by Module; Search by Words; Search Projects; Most Popular. Top Python APIs Popular Projects. Java; Python; JavaScript; TypeScript; C++; Scala; ... imglist=imglist, data_name=data_name, label_name=label_name) if aug_list is None: self.auglist = CreateDetAugmenter(data_shape, **kwargs ... Shuffling our data to solve a learning issue - Python Programming Scikit Learn Machine Learning Tutorial for investing with Python p. 15. In this machine learning tutorial, we're going to cover shuffling our data for learning. One of the problems we have right now is that we're training on, for example, 90% of the data. The problem is that our data frame is in alphabetical order of stocks.
Python Random shuffle() Method - W3Schools The shuffle () method takes a sequence, like a list, and reorganize the order of the items. Note: This method changes the original list, it does not return a new list. Syntax random.shuffle ( sequence, function ) Parameter Values More Examples Example You can define your own function to weigh or specify the result.
utils.shuffle_data_and_labels Example - programtalk.com Reshapes the data from samples of 2D images (N, 28, 28) to linearized samples (N, 784). Also cuts a subset of the data/label-set when nmax is set. shuffle lets us reshuffle the set before cutting.
Shuffle an array in Python - GeeksforGeeks Shuffling a sequence of numbers have always been a useful utility, it is nothing but rearranging the elements in an array. Knowing more than one method to achieve this can always be a plus. Let's discuss certain ways in which this can be achieved. Using shuffle() method from numpy library
Python | Ways to shuffle a list - GeeksforGeeks Method #1 : Fisher-Yates shuffle Algorithm. This is one of the famous algorithms that is mainly employed to shuffle a sequence of numbers in python. This algorithm just takes the higher index value, and swaps it with current value, this process repeats in a loop till end of the list. Python3. import random.
Snorkel Python for Labelling Datasets Programmatically Snorkel is a Python library that is used for data labelling. In this article, we will build an application that labels sentences as a question or not a question using Snorkel. ... Pandas is a Python package that is used for data manipulation and analysis. ... To shuffle our dataset, we use a Python package called random. Let's import the ...
Loading own train data and labels in dataloader using pytorch? # create a dataset like the one you describe from sklearn.datasets import make_classification x,y = make_classification () # load necessary pytorch packages from torch.utils.data import dataloader, tensordataset from torch import tensor # create dataset from several tensors with matching first dimension # samples will be drawn from the first …
Shuffle, Split, and Stack NumPy Arrays in Python - Medium First, split the entire dataset into a training set and a testing set. Second, split the features columns from the target column. For example, split 80% of the data into train and 20% into test, then split the features from the columns within each subset.
How to Shuffle Pandas Dataframe Rows in Python • datagy In order to do this, we apply the sample method to our dataframe and tell the method to return the entire dataframe by passing in frac=1. This instructs Pandas to return 100% of the dataframe. Let's try this out in Pandas: shuffled = df.sample(frac=1) print(shuffled) # 1 Kate Female 95 95 # 5 Kyra Female 85 85
Shuffling Rows in Pandas DataFrames - Towards Data Science The first option you have for shuffling pandas DataFrames is the panads.DataFrame.sample method that returns a random sample of items. In this method you can specify either the exact number or the fraction of records that you wish to sample. Since we want to shuffle the whole DataFrame, we are going to use frac=1 so that all records are returned.
Dataset Splitting Best Practices in Python - KDnuggets Thankfully, the train_test_split module automatically shuffles data first by default (you can override this by setting the shuffle parameter to False ). To do so, both the feature and target vectors ( X and y) must be passed to the module. You should set a random_state for reproducibility.
Pandas Shuffle DataFrame Rows Examples - Spark by {Examples} By using pandas.DataFrame.sample() method you can shuffle the DataFrame rows randomly, if you are using the NumPy module you can use the permutation() method to change the order of the rows also called the shuffle. Python also has other packages like sklearn that has a method shuffle() to shuffle the order of rows in DataFrame. 1. Create a DataFrame with a Dictionary of Lists
Shuffling multiple lists in Python | Wadie Skaf | Towards Dev import random X_train = [40, 60, 80, 20, 10] y_train = [1, 0 ,1 ,0, 1] temp = list(zip(X_train, y_train)) random.shuffle(temp) X_train, y_train = zip(*temp) This simple and beautiful piece of code will shuffle two lists and retain the samples' corresponding order. I hope that by providing this tip, I have made your Python work easier and more enjoyable.
Labeling of data part 1 - Python Programming Tutorials With that, we're looking to now label our data. To do that, we're going to compare the stock's percentage change to the S&P 500's percentage change. If the stock's percent change is less than the S&P 500, then the stock is and under-performing stock. If the percentage change is more, than the label is out-perform.
Post a Comment for "40 shuffle data and labels python"